基本数据类型
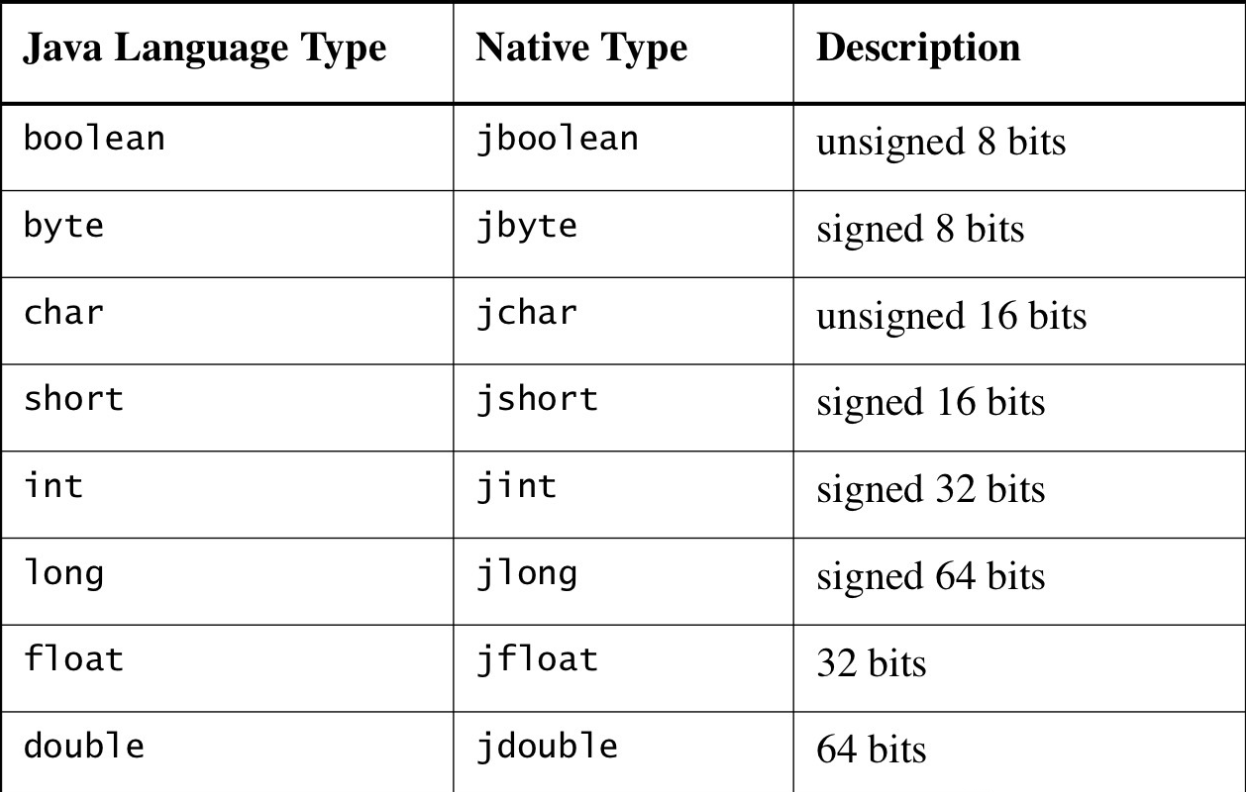
1 2 3 4 5 6 7 8 9 10 11 12
|
#ifndef JNI_TYPES_ALREADY_DEFINED_IN_JNI_MD_H
typedef unsigned char jboolean; typedef unsigned short jchar; typedef short jshort; typedef float jfloat; typedef double jdouble;
typedef jint jsize;
|
接下来,我们找到找到jnt_md.h中的数据定义。 jni_md.h 包含了针对 jbyte、jint 和 jlong 的机器相关的类型定义。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| #ifdef TARGET_ARCH_x86 # include "jni_x86.h" #endif #ifdef TARGET_ARCH_sparc # include "jni_sparc.h" #endif #ifdef TARGET_ARCH_zero # include "jni_zero.h" #endif #ifdef TARGET_ARCH_arm # include "jni_arm.h" #endif #ifdef TARGET_ARCH_ppc # include "jni_ppc.h" #endif
|
那我们继续查看jint_x86.h
:
1 2 3 4 5 6 7 8
| typedef int jint; #if defined(_LP64) typedef long jlong; #else typedef long long jlong; #endif typedef signed char jbyte;
|
引用数据类型
下面是一些引用数据类型,使用typedef定义别名。

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| #ifdef __cplusplus
class _jobject {}; class _jclass : public _jobject {}; class _jthrowable : public _jobject {}; class _jstring : public _jobject {}; class _jarray : public _jobject {}; class _jbooleanArray : public _jarray {}; class _jbyteArray : public _jarray {}; class _jcharArray : public _jarray {}; class _jshortArray : public _jarray {}; class _jintArray : public _jarray {}; class _jlongArray : public _jarray {}; class _jfloatArray : public _jarray {}; class _jdoubleArray : public _jarray {}; class _jobjectArray : public _jarray {};
typedef _jobject *jobject; typedef _jclass *jclass; typedef _jthrowable *jthrowable; typedef _jstring *jstring; typedef _jarray *jarray; typedef _jbooleanArray *jbooleanArray; typedef _jbyteArray *jbyteArray; typedef _jcharArray *jcharArray; typedef _jshortArray *jshortArray; typedef _jintArray *jintArray; typedef _jlongArray *jlongArray; typedef _jfloatArray *jfloatArray; typedef _jdoubleArray *jdoubleArray; typedef _jobjectArray *jobjectArray;
|
弱引用类型
参考链接:https://juejin.cn/post/6934309297815977992
方法签名时用到的公共体类型jvalue
jvalue是一个unio(联合)类型。在C语中为了节约内存,会用联合类型变量来存储声明在联合体中的随意类型数据 。在JNI中将基本数据类型与引用类型定义在一个联合类型中,表示用jvalue定义的变量,能够存储随意JNI类型的数据。原型以下:
1 2 3 4 5 6 7 8 9 10 11
| typedef union jvalue { jboolean z; jbyte b; jchar c; jshort s; jint i; jlong j; jfloat f; jdouble d; jobject l; } jvalue;
|
jfieldID和jMethodID
1 2 3 4 5
| struct _jfieldID; typedef struct _jfieldID *jfieldID;
struct _jmethodID; typedef struct _jmethodID *jmethodID;
|
四种不同引用类型的定义
1 2 3 4 5 6 7
| typedef enum _jobjectType { JNIInvalidRefType = 0, JNILocalRefType = 1, JNIGlobalRefType = 2, JNIWeakGlobalRefType = 3 } jobjectRefType;
|
布尔值
1 2 3 4 5 6
|
#define JNI_FALSE 0 #define JNI_TRUE 1
|
JNI函数的返回值
1 2 3 4 5 6 7 8 9 10 11
|
#define JNI_OK 0 #define JNI_ERR (-1) #define JNI_EDETACHED (-2) #define JNI_EVERSION (-3) #define JNI_ENOMEM (-4) #define JNI_EEXIST (-5) #define JNI_EINVAL (-6)
|
其他常量
1 2 3 4 5 6
|
#define JNI_COMMIT 1 #define JNI_ABORT 2
|
JNI Native Method
1 2 3 4 5
| typedef struct { char *name; char *signature; void *fnPtr; } JNINativeMethod;
|
JNI_ENV变量
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
|
struct JNINativeInterface_;
struct JNIEnv_;
#ifdef __cplusplus typedef JNIEnv_ JNIEnv;
struct JNINativeInterface_{ ........ ........ } struct JNIEnv_ { const struct JNINativeInterface_ *functions; #ifdef __cplusplus
jint GetVersion() { return functions->GetVersion(this); }
|
JavaVM变量
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
|
struct JNIInvokeInterface_;
struct JavaVM_;
#ifdef __cplusplus typedef JavaVM_ JavaVM;
struct JNIInvokeInterface_ { void *reserved0; void *reserved1; void *reserved2;
jint (JNICALL *DestroyJavaVM)(JavaVM *vm);
jint (JNICALL *AttachCurrentThread)(JavaVM *vm, void **penv, void *args);
jint (JNICALL *DetachCurrentThread)(JavaVM *vm);
jint (JNICALL *GetEnv)(JavaVM *vm, void **penv, jint version);
jint (JNICALL *AttachCurrentThreadAsDaemon)(JavaVM *vm, void **penv, void *args); };
struct JavaVM_ { const struct JNIInvokeInterface_ *functions; #ifdef __cplusplus
jint DestroyJavaVM() { return functions->DestroyJavaVM(this); } jint AttachCurrentThread(void **penv, void *args) { return functions->AttachCurrentThread(this, penv, args); } jint DetachCurrentThread() { return functions->DetachCurrentThread(this); }
jint GetEnv(void **penv, jint version) { return functions->GetEnv(this, penv, version); } jint AttachCurrentThreadAsDaemon(void **penv, void *args) { return functions->AttachCurrentThreadAsDaemon(this, penv, args); } #endif };
|
其余的
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| #ifdef _JNI_IMPLEMENTATION_ #define _JNI_IMPORT_OR_EXPORT_ JNIEXPORT #else #define _JNI_IMPORT_OR_EXPORT_ JNIIMPORT #endif _JNI_IMPORT_OR_EXPORT_ jint JNICALL JNI_GetDefaultJavaVMInitArgs(void *args);
_JNI_IMPORT_OR_EXPORT_ jint JNICALL JNI_CreateJavaVM(JavaVM **pvm, void **penv, void *args);
_JNI_IMPORT_OR_EXPORT_ jint JNICALL JNI_GetCreatedJavaVMs(JavaVM **, jsize, jsize *);
JNIEXPORT jint JNICALL JNI_OnLoad(JavaVM *vm, void *reserved);
JNIEXPORT void JNICALL JNI_OnUnload(JavaVM *vm, void *reserved);
#define JNI_VERSION_1_1 0x00010001 #define JNI_VERSION_1_2 0x00010002 #define JNI_VERSION_1_4 0x00010004 #define JNI_VERSION_1_6 0x00010006 #define JNI_VERSION_1_8 0x00010008
#ifdef __cplusplus } #endif
#endif
|
参考
https://blog.csdn.net/qq_36653924/article/details/124470927
https://www.jianshu.com/p/27eaa6669009
https://www.cnblogs.com/cynchanpin/p/7136178.html